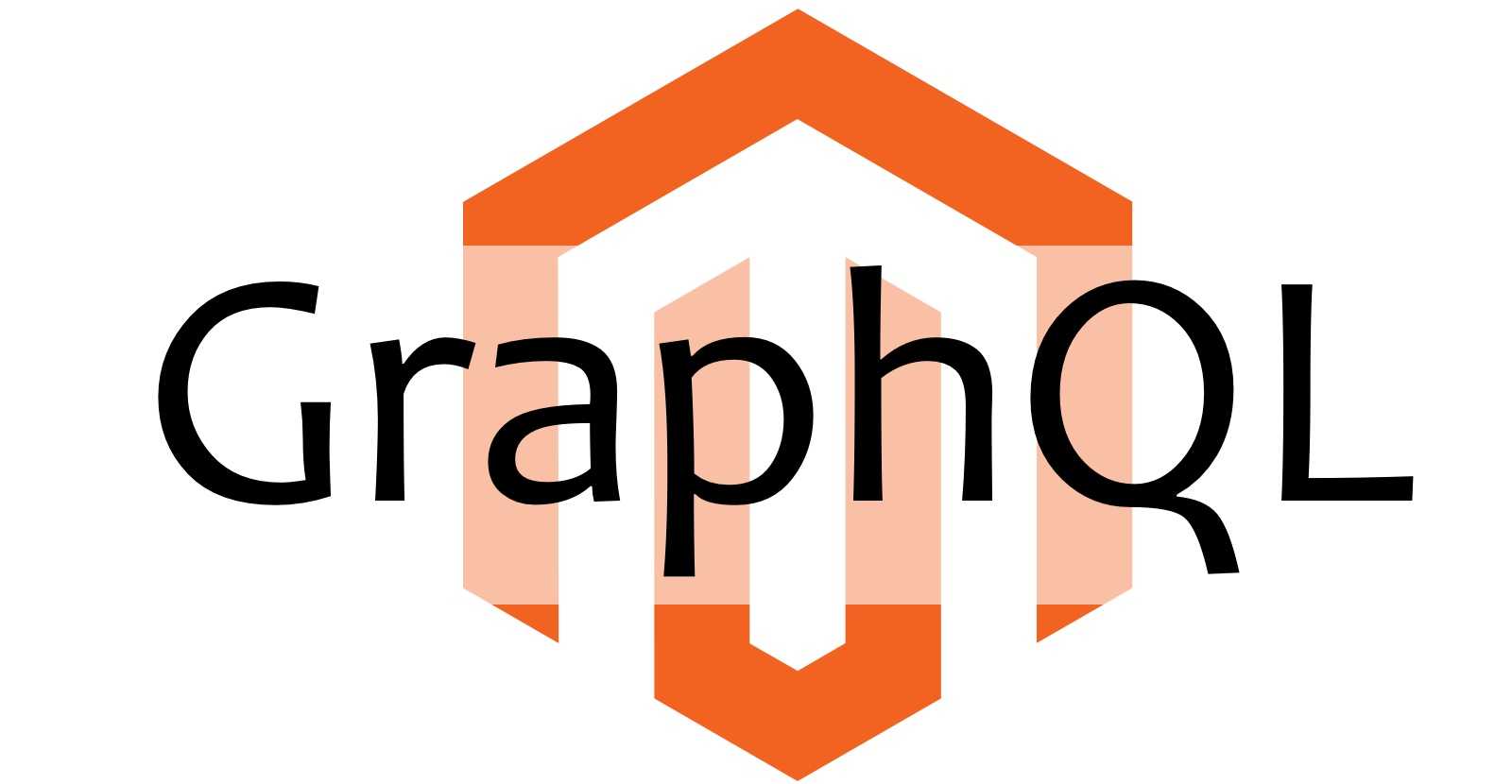
Magento GraphQL - Tips and Tricks
GraphQL is becoming more and more popular and there are quite a few reasons for that. And it’s good that Magento sees this trend and has started work on implementing GraphQL support right in the core.
Enabling GraphQL in Magento
Currently you will have to clone Magento 2.3-develop branch to have GraphQL support. Currently supported are mostly catalog, CMS, and some other small modules. But you can already start building your PWA theme based on that.
Conventions
As you will notice almost instantly, all GraphQL-related modules are separated from the main modules. The reason for that is to have a way to easily disable GraphQL support for any module. Also Magento has added a separate area specifically for GraphQL which is called - graphql.
So if you need to have a separate di.xml, etc for GraphQL area only - you need to put these in etc/graphql/ directory of your module.
Enabling Mutations in Magento GraphQL
In the time of the writing this article, Magento 2.3 didn’t support mutations. And it would be more correct to say — they just weren’t enabled in the schema. Since the underlying library which Magento uses for the whole GraphQL machinery supports mutations just fine.
Back to Magento.. If you would try to define a mutation and run it — you’ll get this error:
Schema is not configured for mutations
But this small issue can’t stop us. To fix it we just go in Magento/GraphQl/Schema/SchemaGenerator.php and change it a bit:
public function generate() : Schema
{
$schema = $this->schemaFactory->create(
[
'query' => $this->outputMapper->getOutputType('Query'),
'mutation' => $this->outputMapper->getOutputType('Mutation'),
'typeLoader' => function ($name) {
return $this->outputMapper->getOutputType($name);
},
'types' => function () {
//all types should be generated only on introspection
$typesImplementors = [];
foreach ($this->config->getDeclaredTypeNames() as $name) {
$typesImplementors [] = $this->outputMapper->getOutputType($name);
}
return $typesImplementors;
}
]
);
return $schema;
}
I’m pretty sure Magento will soon add this little line there, so you can just add a patch to your build script/composer.
Sample GraphQL mutation definition:
type TestQueryResult {
id: String!
}
type Mutation {
testMutation(foo: String!): TestQueryResult
@resolver(class: "Mobelop\\GraphQl\\Model\\Resolver\\TestMutation")
@doc(description: "Test Mutation Resolver")
}
Then you can run it using this query:
mutation {
testMutation(foo:"FOO") {
id
}
}
And the result will be:
{
"data": {
"testMutation": {
"id": "mutation result: FOO"
}
}
}
Working with the GraphQL Endpoint
You can do that through curl surely :D But there are a few nicer tools for this task.
The best one is (in my opinion):
Which looks like this:
Debugging GraphQL Queries
To debug GraphQL you need to enable xdebug in your php.ini and then you can just append ?XDEBUG_SESSION_START=youridekey parameter to your store graphql endpoint like this:
http://yourstore.dev/graphql?XDEBUG_SESSION_START=youridekey
Then configure PHP Remote Debug as usual in your favourite Intellij IDEA or PHPStorm IDE. (See what I did here? and I’m not even being paid for this)
Want to Contribute?
Join #graphql channel on the Community Engineering Slack and ask what you can help with.
Limitations
Right out of the box, Magento GraphQL implementation doesn’t support OPTIONS requests, mutations. And introspection as it is done by default by tools such as GraphQL Playground or GraphiQL is not supported either (so you won’t see your schema there and auto-completion won’t work)